Stack
- Stack is an ordered list in which, insertion and deletion can be performed only at one end that is called top.
- Stack is a recursive data structure having pointer to its top element.
- Stacks are sometimes called as Last-In-First-Out (LIFO) lists i.e. the element which is inserted first in the stack, will be deleted last from the stack.
Applications of Stack
- Recursion
- Expression evaluations and conversions
- Parsing
- Browsers
- Editors
- Tree Traversals
Operations on Stack
There are various operations which can be performed on stack.

1. Push : Adding an element onto the stack
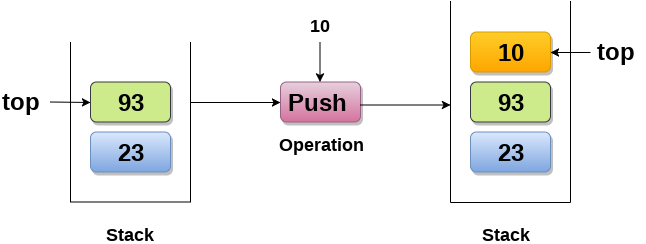
2. Pop : Removing an element from the stack

3. Peek : Look all the elements of stack without removing them.
How the stack grows?
Scenario 1 : Stack is empty
The stack is called empty if it doesn't contain any element inside it. At this stage, the value of variable top is -1.
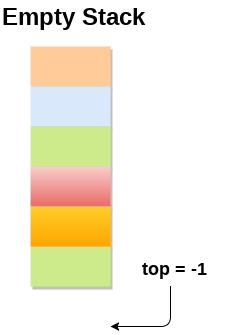
Scenario 2 : Stack is not empty
Value of top will get increased by 1 every time when we add any element to the stack. In the following stack, After adding first element, top = 2.
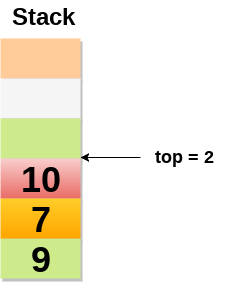
Scenario 3 : Deletion of an element
Value of top will get decreased by 1 whenever an element is deleted from the stack.
In the following stack, after deleting 10 from the stack, top = 1.

Top and its value :
Top position | Status of stack |
---|---|
-1 | Empty |
0 | Only one element in the stack |
N-1 | Stack is full |
N | Overflow |